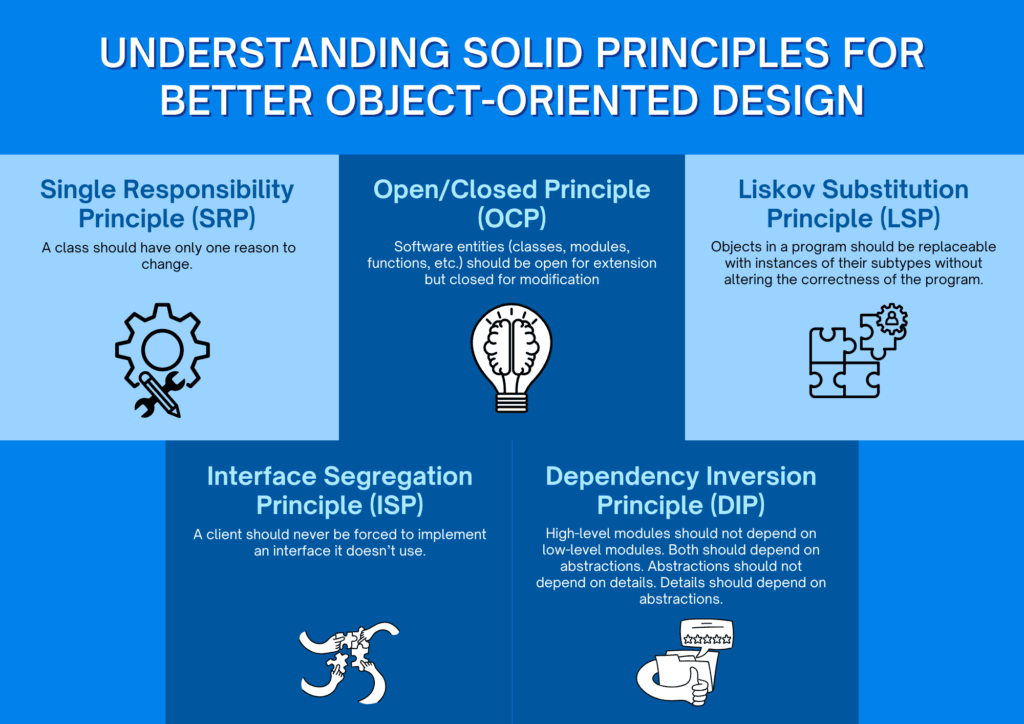
In the world of software development, designing clean, scalable, and maintainable systems is critical to the long-term success of any project. Whether you’re building a small application or a large, enterprise-level system, adhering to a solid design structure is essential to ensure your codebase remains easy to understand, modify, and extend over time. This is where SOLID principles come into play.
The SOLID principles are a set of guidelines that help software engineers design systems that are easy to maintain, flexible, and scalable. These principles form the foundation for good object-oriented design and promote best practices that minimize the risk of code rot, technical debt, and future maintenance headaches.
In this blog post, we’ll explore each of the SOLID principles in depth and show how applying these concepts can improve the overall design of your object-oriented systems.
What Are the SOLID Principles?
The acronym SOLID represents five principles of object-oriented design that were introduced by Robert C. Martin (a.k.a. Uncle Bob). These principles provide a structured approach to designing software that is modular, maintainable, and scalable.
Here’s a quick overview of what each letter in SOLID stands for:
- S – Single Responsibility Principle (SRP)
- O – Open/Closed Principle (OCP)
- L – Liskov Substitution Principle (LSP)
- I – Interface Segregation Principle (ISP)
- D – Dependency Inversion Principle (DIP)
Now, let’s dive deeper into each of these principles and examine how they contribute to better software design.
1. Single Responsibility Principle (SRP)
Definition: A class should have only one reason to change.
The Single Responsibility Principle (SRP) states that a class should only have one responsibility or reason for change. In other words, a class should focus on doing one thing and doing it well. This ensures that when changes are needed in one part of the functionality, you won’t inadvertently affect other unrelated parts.
Why SRP Matters:
When a class has multiple responsibilities, any change to one responsibility could potentially impact the other. This leads to code that is harder to maintain, debug, and test. By following SRP, you ensure that each class remains small and focused, making the codebase easier to manage and more resilient to changes.
Example:
class ReportGenerator:
def generate_report(self):
# Logic for generating a report
pass
def send_report(self):
# Logic for sending the report
pass
In this example, the ReportGenerator
class violates SRP because it is responsible for both generating and sending reports. Instead, you should separate these responsibilities:
class ReportGenerator:
def generate_report(self):
# Logic for generating a report
pass
class ReportSender:
def send_report(self):
# Logic for sending the report
pass
Now, each class has a single responsibility: one for generating the report and another for sending it.
2. Open/Closed Principle (OCP)
Definition: Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
The Open/Closed Principle (OCP) means that your classes or modules should be designed in such a way that new functionality can be added without modifying existing code. In essence, you should be able to extend a class’s behavior without altering its source code, which helps avoid introducing bugs.
Why OCP Matters:
The more you modify existing code, the greater the chance you’ll introduce bugs into a previously working system. OCP encourages a design that allows new features to be added through inheritance or composition without altering existing behavior.
Example:
class Invoice:
def calculate_discount(self, customer_type):
if customer_type == "regular":
return 0.1
elif customer_type == "premium":
return 0.2
This code violates OCP because every time a new customer type is introduced, the Invoice
class has to be modified. Instead, we can refactor the code to adhere to OCP by using inheritance or strategy design patterns:
class Discount:
def calculate_discount(self):
raise NotImplementedError
class RegularCustomerDiscount(Discount):
def calculate_discount(self):
return 0.1
class PremiumCustomerDiscount(Discount):
def calculate_discount(self):
return 0.2
Now, new types of discounts can be added without modifying the existing discount classes.
3. Liskov Substitution Principle (LSP)
Definition: Objects in a program should be replaceable with instances of their subtypes without altering the correctness of the program.
The Liskov Substitution Principle (LSP) ensures that derived classes or subclasses can be used in place of their base classes without affecting the functionality of the system. In other words, subclasses should be able to stand in for the parent class and behave in the same manner without introducing bugs or unexpected behaviors.
Why LSP Matters:
Violating LSP can lead to broken inheritance hierarchies where the derived classes don’t fully adhere to the behavior of the base class. This can result in unpredictable outcomes when using polymorphism.
Example:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Square(Rectangle):
def __init__(self, side):
super().__init__(side, side)
In this example, the Square
class is a subclass of Rectangle
, but it violates LSP because a square is not a type of rectangle in this context, as changing the width or height of a rectangle should not affect both dimensions. To adhere to LSP, you can separate these classes:
class Shape:
def area(self):
raise NotImplementedError
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Square(Shape):
def __init__(self, side):
self.side = side
def area(self):
return self.side * self.side
Now, Rectangle
and Square
are independent, and the substitution will work without issues.
4. Interface Segregation Principle (ISP)
Definition: A client should never be forced to implement an interface it doesn’t use.
The Interface Segregation Principle (ISP) suggests that instead of creating large, monolithic interfaces, you should break them down into smaller, more specific ones. This ensures that classes only need to implement the methods that are relevant to them.
Why ISP Matters:
Large interfaces can force classes to implement methods they don’t need, which results in unnecessary code and complexity. By keeping interfaces small and focused, you ensure that implementations remain clean and relevant.
Example:
class Worker:
def work(self):
pass
def eat(self):
pass
If there are workers that don’t need to implement the eat
method (e.g., robots), they are still forced to do so. Instead, you can split the interfaces:
class Workable:
def work(self):
pass
class Eatable:
def eat(self):
pass
Now, different types of workers can implement only the methods they need.
5. Dependency Inversion Principle (DIP)
Definition: High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
The Dependency Inversion Principle (DIP) promotes decoupling by ensuring that high-level modules don’t directly depend on low-level implementations. Instead, both should depend on abstractions (such as interfaces or abstract classes). This reduces the risk of tight coupling between components, making the system more flexible and scalable.
Why DIP Matters:
When high-level modules directly depend on low-level implementations, any change to the low-level code requires changes to the high-level code as well. By using abstractions, you can make changes to one part of the system without affecting others.
Example:
class MySQLDatabase:
def connect(self):
# Connection logic
pass
class Application:
def __init__(self):
self.database = MySQLDatabase()
In this example, Application
is tightly coupled to MySQLDatabase
. Instead, we can introduce an abstraction:
pythonCopy codeclass Database:
def connect(self):
pass
class MySQLDatabase(Database):
def connect(self):
# Connection logic
pass
class Application:
def __init__(self, database: Database):
self.database = database
Now, Application
depends on an abstraction (the Database
interface), allowing you to switch to a different database without modifying the Application
class.
Conclusion
The SOLID principles offer a clear path to building well-structured, maintainable, and scalable object-oriented systems. By adhering to these principles, you can design systems that are easier to understand, extend, and maintain over time, reducing the likelihood of encountering technical debt or bugs.
To recap, the SOLID principles are:
- S – Single Responsibility Principle
- O – Open/Closed Principle
- L – Liskov Substitution Principle
- I – Interface Segregation Principle
- D – Dependency Inversion Principle
By applying these principles, you can improve the quality and longevity of your software projects. So, whether you’re working on a new project or refactoring an existing codebase, keep these principles in mind to create better, more maintainable software.