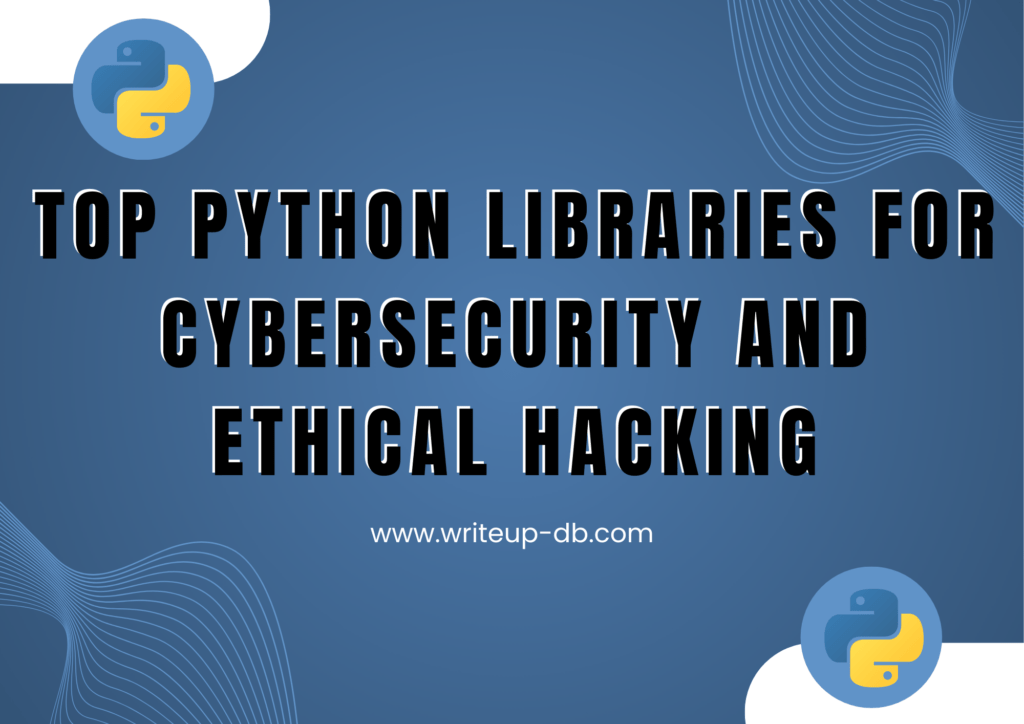
Python has rapidly become one of the go-to programming languages for cybersecurity professionals and ethical hackers. Its simplicity, readability, and vast array of libraries make it the ideal language for writing scripts, automating tasks, and conducting various penetration testing activities. Whether you’re a beginner just starting your ethical hacking journey or an experienced cybersecurity expert, Python’s extensive library ecosystem can be a powerful ally in identifying and mitigating security risks.
In this blog post, we will dive into some of the top Python libraries for cybersecurity and ethical hacking that you should know about. These libraries will help you perform everything from network scanning to password cracking, web scraping, and vulnerability testing.
1. Scapy
Purpose: Packet Manipulation and Network Scanning
Why it’s essential: Scapy is a powerful Python library used for network packet manipulation. It allows you to forge and decode packets, capture traffic, probe networks, and create custom packet filters. Whether you’re analyzing network traffic or writing your own network protocol implementation, Scapy is one of the best tools to have in your toolkit.
Features:
- Packet sniffing and packet injection
- Network discovery and scanning (ARP, ICMP, etc.)
- Attacks on wireless networks (WiFi de-authentication attacks)
- DNS spoofing, ARP poisoning, and more
Example Use Case:
from scapy.all import *
# Sending a simple ping request
packet = IP(dst="192.168.1.1")/ICMP()
response = sr1(packet)
print(response.show())
2. Nmap (Python-Nmap)
Purpose: Network Scanning and Vulnerability Detection
Why it’s essential: Nmap is one of the most widely used network scanning tools. The Python-Nmap library allows you to automate Nmap scanning from within your Python scripts. This library enables security professionals to perform network discovery, port scanning, OS fingerprinting, and vulnerability detection.
Features:
- Automates Nmap scans through Python
- Can run multiple scan types like TCP/UDP scans, version detection, and OS detection
- Easy integration with other Python programs and scripts
Example Use Case:
import nmap
scanner = nmap.PortScanner()
scanner.scan('192.168.1.0/24', '22-443')
for host in scanner.all_hosts():
print(f"Host: {host} ({scanner[host].hostname()})")
print(f"State: {scanner[host].state()}")
3. Requests
Purpose: Web Requests and API Interaction
Why it’s essential: The Requests library is incredibly useful when dealing with web-based attacks, such as sending HTTP requests, automating interactions with web services, or testing APIs for security vulnerabilities. This library makes it easy to send all types of HTTP requests and handle responses.
Features:
- Supports GET, POST, PUT, DELETE, and other HTTP methods
- Built-in support for handling cookies, sessions, and redirections
- Perfect for web scraping and penetration testing of web apps
Example Use Case:
import requests
response = requests.get('http://example.com')
if response.status_code == 200:
print("Website is accessible!")
4. Paramiko
Purpose: SSH Communication and Remote Access
Why it’s essential: Paramiko is a Python library for handling SSH connections, which can be useful for automating tasks such as remote command execution, file transfers, and performing actions on remote servers. It’s especially useful in environments where secure communication is essential for testing and maintaining infrastructure.
Features:
- Secure shell access to remote systems
- Supports both client and server-side operations
- Can be used to automate tasks such as file transfers, backups, and command execution
Example Use Case:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('192.168.1.100', username='user', password='password')
stdin, stdout, stderr = ssh.exec_command('ls')
print(stdout.read().decode())
ssh.close()
5. BeautifulSoup
Purpose: Web Scraping and HTML Parsing
Why it’s essential: BeautifulSoup is one of the most popular libraries for web scraping. It helps you parse and extract information from HTML and XML documents. For cybersecurity professionals, BeautifulSoup can be used for collecting data from vulnerable web applications, extracting links, identifying hidden fields, and more.
Features:
- Parses HTML and XML documents efficiently
- Handles malformed HTML gracefully
- Works well with other libraries like Requests and Scrapy for automated scraping
Example Use Case:
from bs4 import BeautifulSoup
import requests
response = requests.get('http://example.com')
soup = BeautifulSoup(response.content, 'html.parser')
# Extract all the links from the webpage
for link in soup.find_all('a'):
print(link.get('href'))
6. PwnTools
Purpose: Exploit Development and Reverse Engineering
Why it’s essential: PwnTools is a Python library designed specifically for CTF (Capture the Flag) competitions and exploit development. It provides a set of useful functions for tasks like generating payloads, interacting with remote servers, and automating exploits.
Features:
- Automatically generates shellcode and ROP (Return Oriented Programming) chains
- Works well with binary exploitation and reverse engineering challenges
- Useful for both beginners and advanced users working on exploit development
Example Use Case:
from pwn import *
p = remote('example.com', 1234)
p.sendline('payload')
response = p.recv()
print(response)
7. Cryptography
Purpose: Encryption, Decryption, and Secure Communication
Why it’s essential: Cryptography is a crucial part of cybersecurity, and this library makes it easy to work with cryptographic algorithms such as AES, RSA, and SHA. Whether you’re encrypting data, verifying signatures, or building secure communications, the Cryptography library provides the necessary tools to achieve it securely.
Features:
- Support for symmetric and asymmetric encryption
- Provides a simple and high-level API for cryptographic operations
- Handles hashing, key management, and digital signatures
Example Use Case:
from cryptography.fernet import Fernet
key = Fernet.generate_key()
cipher_suite = Fernet(key)
cipher_text = cipher_suite.encrypt(b"Sensitive Data")
plain_text = cipher_suite.decrypt(cipher_text)
print(plain_text.decode())
8. Shodan
Purpose: Internet-Connected Device Search
Why it’s essential: Shodan is known as the “search engine for hackers” as it allows you to find Internet-connected devices worldwide. The Shodan Python library allows you to automate searches and queries against the Shodan database, which can be useful for vulnerability scanning and security research.
Features:
- Finds connected devices and services on the Internet
- Can help identify exposed services like webcams, servers, or industrial control systems
- Useful for open-source intelligence (OSINT) and reconnaissance
Example Use Case:
import shodan
api = shodan.Shodan('YOUR_API_KEY')
results = api.search('apache')
for result in results['matches']:
print(f"IP: {result['ip_str']} | Port: {result['port']}")
9. Pwntools
Purpose: Exploit Development and CTF Challenges
Why it’s essential: Pwntools is another powerful tool for CTF challenges and exploit development. With this library, you can build and test binary exploits, craft shellcode, perform heap and stack exploits, and even interface with remote servers in a CTF environment.
Features:
- Great for buffer overflow attacks and binary exploitation
- Helps build shellcode and format strings
- Integrates well with network-based and process-based challenges in CTFs
10. Twisted
Purpose: Networking and Asynchronous Programming
Why it’s essential: Twisted is a powerful Python library for networking. It allows you to build scalable and asynchronous network servers, which can be useful for developing your own tools or for simulating attack scenarios. Twisted supports protocols like HTTP, DNS, and SSH.
Features:
- Asynchronous I/O for handling multiple connections
- Ideal for writing network servers and clients
- Supports many different application layer protocols
Example Use Case:
from twisted.internet import reactor, protocol
class Echo(protocol.Protocol):
def dataReceived(self, data):
self.transport.write(data)
class EchoFactory(protocol.Factory):
def buildProtocol(self, addr):
return Echo()
reactor.listenTCP(8000, EchoFactory())
reactor.run()
Conclusion
Python’s rich ecosystem of libraries makes it an invaluable tool for cybersecurity professionals and ethical hackers. Whether you need to perform network scanning, create exploits, interact with web services, or encrypt sensitive data, Python has a library to suit your needs. As you grow in your cybersecurity career, becoming proficient with these libraries can enhance your ability to perform effective penetration testing and security audits, making you more efficient and successful in your role.
By mastering these Python libraries, you’ll be well-equipped to automate tasks, discover vulnerabilities, and develop custom tools to improve cybersecurity defences. Whether you are preparing for a bug bounty program or participating in a CTF challenge, Python can be your ultimate weapon in cybersecurity.
Explore Further:
- Learn More Python: Python.org
- Bug Bounty Platforms: Check out our article on “Top 11 Bug Bounty Platforms”
- CTF Competitions: Participate in Capture The Flag (CTF) events to sharpen your skills!
Happy Hacking!